2.1. Step Testing#
This notebook guides you through the steps necessary to perform a simple step test of a heater/sensor unit on the Temperature Contnrol Lab.
Download this notebook to your laptop, then run these cells to capture step test data.
import matplotlib.pyplot as plt
import pandas as pd
from tclab import TCLab, clock, Historian, Plotter
2.1.1. Executing the Step Test#
2.1.1.1. Verify an Initial Steady State#
Don’t start a step test without first letting the device come to an initial steady state!
A step test assumes the system is initially at steady state. In the case of the Temperature Control Lab, the initial steady with no power input would be room temperature. It generally takes 10 minutes or more to reach steady state. We’ll do a measurement to confirm the initial temperature. Repeat this until you are sure you are at an initial steady state.
lab = TCLab()
print(lab.T1, lab.T1)
lab.close()
TCLab version 0.4.10dev
Arduino Leonardo connected on port /dev/cu.usbmodem143101 at 115200 baud.
TCLab Firmware 2.0.1 Arduino Leonardo/Micro.
23.154 23.154
TCLab disconnected successfully.
2.1.1.2. Conduct the Experiment#
# experimental parameters
P1 = 200
Q1 = 50
tfinal = 600
# perform experiment
with TCLab() as lab:
lab.P1 = P1
h = Historian(lab.sources)
p = Plotter(h, tfinal)
lab.Q1(Q1)
for t in clock(tfinal):
p.update(t)
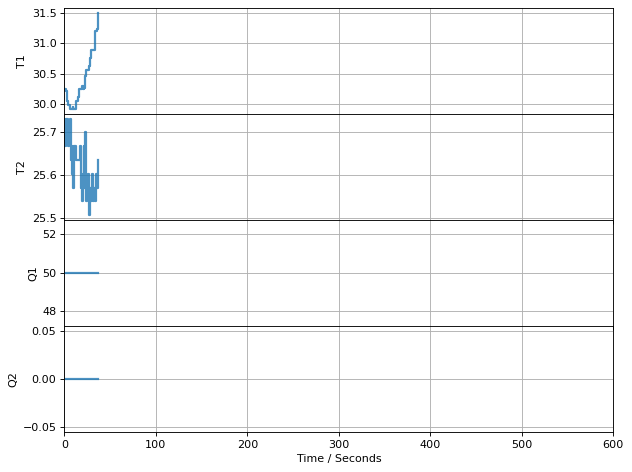
TCLab disconnected successfully.
---------------------------------------------------------------------------
KeyboardInterrupt Traceback (most recent call last)
/var/folders/cm/z3t28j296f98jdp1vqyplkz00000gn/T/ipykernel_26229/1996149352.py in <module>
10 p = Plotter(h, tfinal)
11 lab.Q1(Q1)
---> 12 for t in clock(tfinal):
13 p.update(t)
~/Google Drive/GitHub/TCLab/tclab/labtime.py in clock(period, step, tol, adaptive)
107 '({:.2f} too long). Consider increasing step.')
108 raise RuntimeError(message.format(step, elapsed, elapsed-step))
--> 109 labtime.sleep(step - (labtime.time() - start) % step)
110 now = labtime.time() - start
~/Google Drive/GitHub/TCLab/tclab/labtime.py in sleep(self, delay)
39 self.lastsleep = delay
40 if self._running:
---> 41 time.sleep(delay / self._rate)
42 else:
43 raise RuntimeWarning("sleep is not valid when labtime is stopped.")
KeyboardInterrupt:
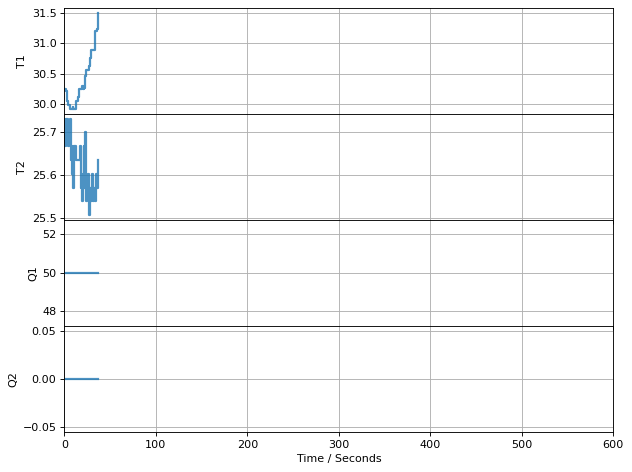
2.1.1.3. Verify the Experimental Data#
h.to_csv("data.csv")
2.1.1.4. Verify the Data File#
import pandas as pd
df = pd.read_csv('data.csv').set_index('Time')
df.plot(grid=True)
<AxesSubplot:xlabel='Time'>
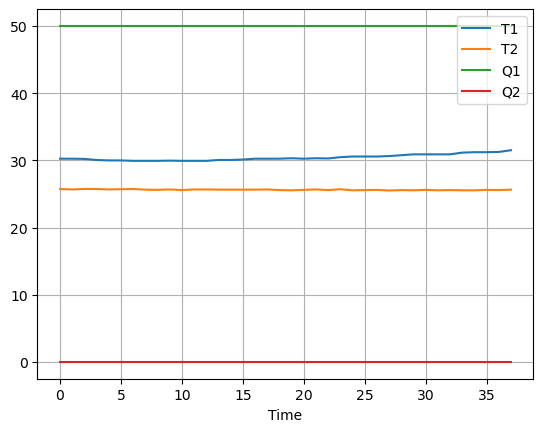
df.head()
T1 | T2 | Q1 | Q2 | |
---|---|---|---|---|
Time | ||||
0.0 | 30.244 | 25.732 | 50.0 | 0.0 |
1.0 | 30.244 | 25.668 | 50.0 | 0.0 |
2.0 | 30.212 | 25.732 | 50.0 | 0.0 |
3.0 | 30.051 | 25.732 | 50.0 | 0.0 |
4.0 | 29.986 | 25.668 | 50.0 | 0.0 |
df.tail()
T1 | T2 | Q1 | Q2 | |
---|---|---|---|---|
Time | ||||
33.0 | 31.146 | 25.539 | 50.0 | 0.0 |
34.0 | 31.211 | 25.539 | 50.0 | 0.0 |
35.0 | 31.211 | 25.604 | 50.0 | 0.0 |
36.0 | 31.243 | 25.571 | 50.0 | 0.0 |
37.0 | 31.501 | 25.636 | 50.0 | 0.0 |